As we know, class is a way to represent any entity. Inside that, there are variables that store the information related to that entity. And, are also some functions that store the methods to modify and control the real-world entity.
For example, we have to represent a car. For this, we will make the car class and inside that, there are variables such as price, fuel type, engine, color, etc. to store the necessary information related to a car.
Also, there will be some methods to control some functioning while making the car, such as setColor(), setAlarm(), etc.
Now, it is required to make a class to represent another real-world entity, that is somehow related to the previous class that we have created. For example, now we have to represent Sports Car. As we know that sports car will be somehow related to a car, or it will be a car with some more special functions.
So, while making the class, we will need to add some more new functions and variables. And, we will also need to make those variables that are already available to us in the car class. This will lead to extra unnecessary work.
To prevent unnecessary load, we have the concept of Inheritance in Python.
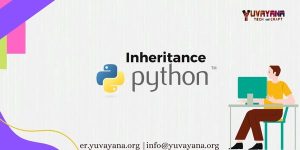
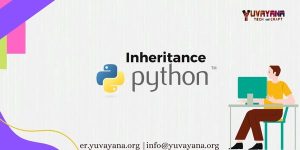
Given below is a Program through which we will understand this concept better –
class Person(object): def __init__(self, name): self.name = name def getName(self): return self.name def isEmployee(self): return False class Employee(Person): def isEmployee(self): return True emp = Person("Yuvayana") print(emp.getName(), emp.isEmployee()) emp = Employee("Tech") print(emp.getName(), emp.isEmployee())
Output –
Yuvayana False Tech True
Issubclass() function
Python provides the issubclass() function that tells us directly if a class is a subclass of another class
class Base(object): pass class Derived(Base): pass print(issubclass(Derived, Base)) print(issubclass(Base, Derived)) d = Derived() b = Base() print(isinstance(b, Derived)) print(isinstance(d, Base))
Output –
True False False True
Method Overriding in Python
When we re-create the same method in the child class, this refers to method overriding.
For method overriding the following two conditions should be met-
First, there should be inheritance. Because function overriding cannot be done within a class.
And second, The function re-defined in the child class should have the same number of parameters
Further, we will understand inheritance with the help of the example given below –
class Animal: multicellular = True eukaryotic = True def breathe(self): print("I breathe oxygen.") def feed(self): print("I eat food.")
Now, we will create a child class herbivorous which will extend the class animal –
class Herbivorous(Animal): #function feed def feed(self): print("I eat only plants. I am vegetarian.")
In the child class Herbivorous, we have overridden the method feed().
So now when we create an object of the class Herbivorous and call the method feed() the overridden version will be executed.
herbi = Herbivorous() herbi.feed() herbi.breathe()
Output –
I eat only plants. I am vegetarian. I breathe oxygen.
So, in this article, we have learned about one of the very useful concepts of Object Oriented programming which is Inheritance. This is used quite often when we are working on a project where a task is divided among multiple team members.
Attempt Free Python Online Test